Introduction to Scripting¶
This detailed guide describes how to write Ruby scripts to automate tasks in Datavyu and to ensure reliable coding.
Classes, Methods, and Parameters provides an overview of the methods and classes of the Datavyu API. For a more in-depth discussion of each particular method and function, refer to the Reference documentation.
Recommended Text Editors¶
Ruby scripts are simply text files with a .rb
file extension. You
can write scripts in any text editor, including built-in ones like
Notepad or TextEdit. However, while those programs are adequate for
scripting purposes, modern text editors make scripts much easier to
read by providing syntax highlighting, which can make a world of
difference when attempting to debug an issue.
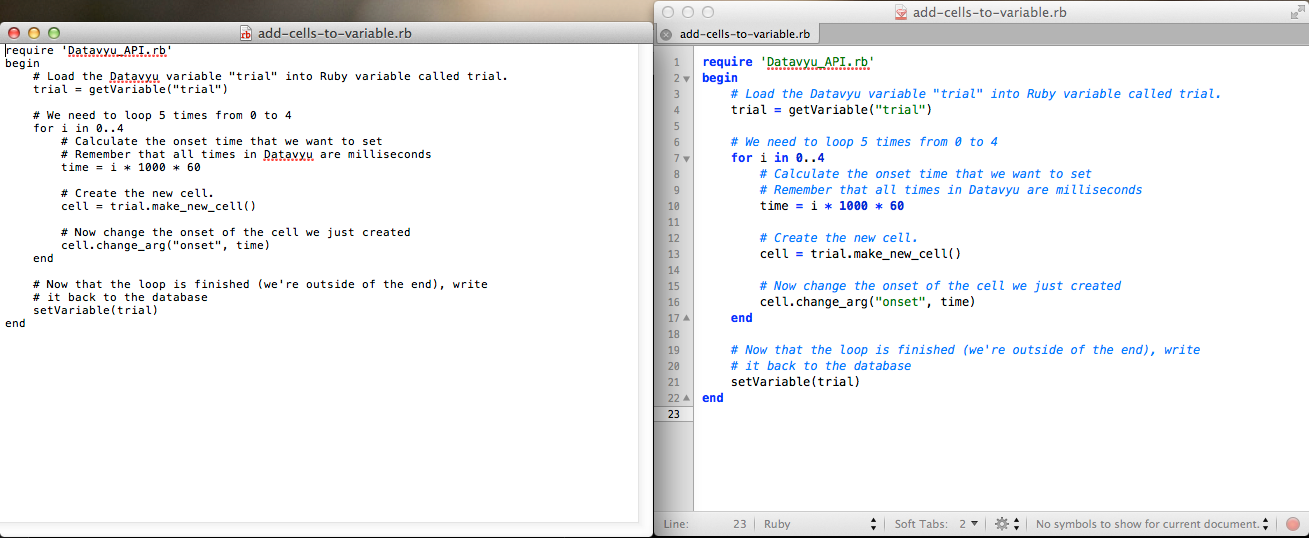
At left: TextEdit (Mac OS X). At right: TextMate 2 (Mac OS X) with “Mac
Classic” theme. Note that add-cells-to-variable.rb
is open in
each editor.¶
To take advantage of syntax highlighting and coding support, we recommend installing and using one of the following programs for editing Ruby scripts:
Windows:
Mac OSX:
TextMate 2 (free and open-source)
gedit (free and open-source)
TextWrangler (free)
Linux:
Tip
Most text editors determine what kind of syntax highlighting to use based on the file extension. Please ensure that your Ruby script files end with the “.rb” extension so you see syntax highlighting.
General Principles¶
Each cell that you create with the Datavyu API has three inherent
codes: onset
, offset
, and ordinal
. Each
cell also has at least one user-specified code.
onset
, offset
, and ordinal
are all Integers, while the user-specified codes are Strings.
onset
and offset
are measured in milliseconds from the
beginning of the video, starting from 0. For
instance, an onset
time of 00:02:20 translates to 140000ms.
Since user-specified codes are strings, you must convert any codes that you wish to perform calculations on to a numeric type. This is easily done with Ruby using the to_i method for integers, or the to_f method for floating point numbers.
Example
Create a variable, var1
whose value is “5”. Since the number 5 has
quotation marks around it, it is a string.
var1 = "5"
If you print var
, you’d see that it is “5”. Create a new
variable, var2
from var1
using to_i
to convert the “5” to
a 5
.
var2 = var1.to_i
Print var2
and see that it is a 5
without quotation marks:
print var2
Tip
Ruby provides two options for printing to the console: the p
command and the puts
command. When in doubt, use p
, as it
prints arrays and lists in a more readable format, rather than
mashing them all together like puts
does. Try printing a list
such as [5, 6, 7, 8, 9]
using both p
and puts
to
see the difference.
p [5,6,7,8,9]
puts [5,6,7,8,9]
Basic Script Format¶
Tip
Scripts are very sensitive! When programming, every quotation mark, underscore, period, and slash serve a purpose. You must use the correct syntax or the script will not work.
Code and column names are also case sensitive. If you have a column in a spreadsheet called “trial”, requesting “Trial” will not work.
All code names in Ruby must be lowercase. Codes with uppercases have special meanings.
All Datavyu API scripts must include the following line at the top:
require 'Datavyu_API.rb'
This require
statement loads all of the helper functions that enable
your scripts to interact with the Datavyu spreadsheet.
In general, the rest of the script code goes between begin
and
end
tags, making the general format as follows:
require 'Datavyu_API.rb'
begin
# Get the columns that we want to work with
# Do something to those columns
# Write any changes to those columns back to the spreadsheet
end
Tip
Anything that comes after a #
character on a line in Ruby is a
comment, which means it will not execute any specific task. Comments are useful for
leaving notes that explain what the code is doing so that when you return to an old script
you remember what you’re looking at. The examples in this documentation
use comments extensively.
Now that you are grounded in the Datavyu Ruby API mechanics, consider the API Tutorials or Datavyu Ruby API Reference.